Python3网络库Requests(一)
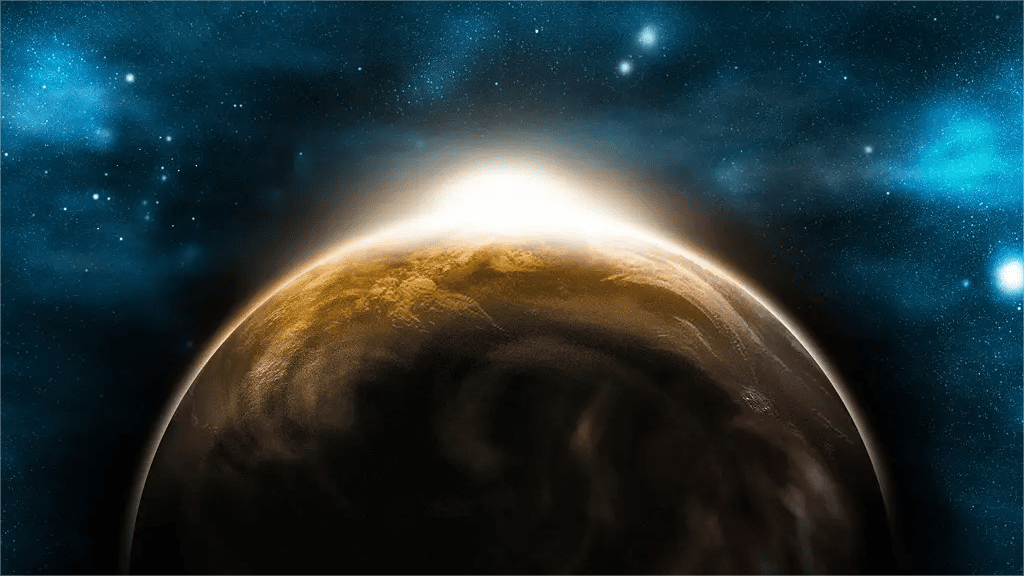
Python3网络库Requests(一)
繁华落尽丶千目前在学习Python3爬虫技术,学习爬虫不仅要熟悉Python的基本知识,还要熟悉其他的库。例如:网络请求库Request,数据解析库Beautifulsoup,lxml,和爬虫框架Scrapy等。本文将学习Requests库的基础知识,后续将学习其他内容。少年加油吧!
版本:Python3.6 Requests2.18.1
Requests安装
笔者使用Mac电脑,至于Windows电脑自行Google。Mac终端运行:
1 | pip install requests |
Mac 系统自带Python2.x,需要自行安装Python3。在Python3环境下安装需要使用
pip3 install requests
Request基础知识
确定前面的内容顺利完成,按我们开始学习如何使用Request吧。
发送一个网络请求
打开终端,运行python3
进入Python模式。
导入模块:
1 | >>> import requests |
发送Get请求
1 | >>> r = requests.get('https://api.github.com/events') |
我们获取了名为r的Response对象,包含了我们需要的所有信息。
发送Post请求
1 | >>> r = requests.post('http://httpbin.org/post', data = {'key':'value'}) |
另外还可以发送Put,Delete,Head和Options等请求
1 | >>> r = requests.put('http://httpbin.org/put', data = {'key':'value'}) |
在URL中传递数据
上面知道如何发送简单网络请求,那么如何在URL中传递参数呢。
Request允许使用params关键字将参数以字符串字典的形式添加。
1 | # 传递参数 |
返回值内容
之前我们获取的r
Response对象中,具体包含什么内容呢。
text
1 | >>> r = requests.get('https://api.github.com/events') |
服务器内容,大多数Unicode字符集都被无缝解码。
encoding
在发送请求时,会对返回值text进行编码猜测,猜测编码类型。使用encoding
可以查看和修改编码类型
1 | >>> r.encoding |
content
将返回正文作为字节,而非文本请求
1 | >>> r.content |
json()
1 | >>> r.json() |
如果JSON解码异常会报
ValueError
错误。有时调用JSON成功并不能证明请求成功,需要使用status_code
进行验证。
raw
如果希望从服务器获取原始socket响应可以使用raw
1 | >>> r.raw |
status_code/raise_for_status
响应状态码
1 | >>> r.status_code |
headers
1 | >>> r.headers |
获取请求头信息
自定义请求头
如果需要向请求添加请求头,只需向headers参数传递一个字典就可以。
1 | >>> url = 'https://api.github.com/some/endpoint' |
更复杂的POST请求
发送JSON参数
1 | >>> url = 'https://api.github.com/some/endpoint' |
使用JSON来代替dict,直接发送未编码的参数。
发送多部分编码文件
1 | >>> url = 'http://httpbin.org/post' |
重定向和历史
history
跟踪重定向
allow_redirects
设置是否允许重定向
请求超时
**timeout **
1 | >>> requests.get('http://github.com', timeout=0.001) |
超时不是整个响应的下载的时间限制,而是服务器的响应时间。
小结
本文讲解最基本的Requests用法,对于刚入门的新手来说暂时够使用。关于Requests的高级功能,随着学习的深入在学习。接下来我们学习Beautifulsoup。